Artificial Intelligence (AI) refers to the simulation of human intelligence in machines that are designed to think and act like humans. It encompasses various technologies, including machine learning (ML), natural language processing (NLP), computer vision, and more. Artificial intelligence allows machines to match, or even improve upon, the capabilities of the human mind. Artificial intelligence aims to provide machines with similar processing and analysis capabilities as humans, making AI a useful counterpart to people in everyday life. AI is able to interpret and sort data at scale, solve complicated problems and automate various tasks simultaneously, which can save time and fill in operational gaps missed by humans.
How Does AI Work?
AI works through algorithms and models that analyze data, learn patterns, and make predictions or decisions. Here’s a simplified breakdown:
1. Data Collection: AI requires data to learn. This data can come from various sources, such as text, images, or structured datasets.
2. Preprocessing: Data is cleaned and formatted to make it suitable for analysis.
3. Model Training: Algorithms learn from the data. This involves adjusting parameters to minimize errors in predictions.
4. Inference: The trained model is used to make predictions or decisions based on new data.
5. Evaluation: The model’s performance is assessed, often using metrics like accuracy or precision.
How to Learn AI: A Beginner's Path
1. Mathematics and Statistics: Understanding basic concepts in linear algebra, calculus, and statistics is essential.
2. Programming: Learn Python, which is widely used in AI. Familiarize yourself with libraries like NumPy, Pandas, and Matplotlib.
3. Machine Learning: Start with introductory courses or books. Focus on algorithms like linear regression, decision trees, and neural networks.
4. Hands-on Projects: Apply your knowledge through small projects. For example, you can create a simple classifier using a dataset.
Basic Code Example: Simple Linear Regression in Python
Here's a simple example of a linear regression model using Python and the scikit-learn library:
python
# Import necessary libraries
import numpy as np
import matplotlib.pyplot as plt
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
# Sample data (e.g., hours studied vs. scores)
X = np.array([[1], [2], [3], [4], [5]]) # Hours studied
y = np.array([1, 2, 3, 4, 5]) # Scores
# Split data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=0)
# Create and train the model
model = LinearRegression()
model.fit(X_train, y_train)
# Make predictions
predictions = model.predict(X_test)
# Visualize the results
plt.scatter(X, y, color='blue')
# Original data
plt.plot(X, model.predict(X), color='red') # Regression line
plt.xlabel('Hours Studied')
plt.ylabel('Scores')
plt.title('Linear Regression Example')
plt.show()
# Print predictions
print("Predictions:", predictions)
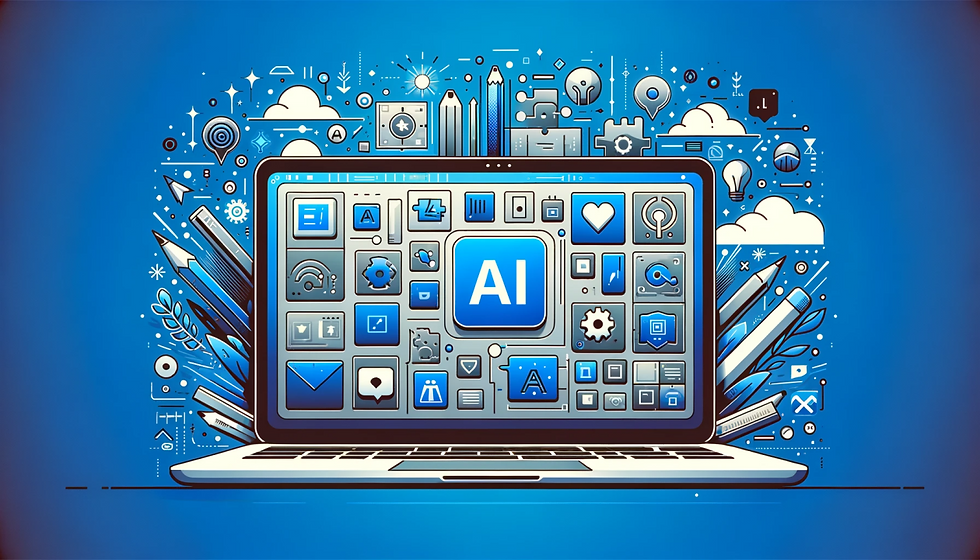
Comments